The wrapper widget for RenderWorld. More...
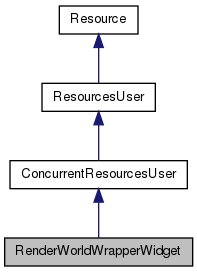
Public Slots | |
bool | isSelfUpdate () |
return true if the self updating is active | |
void | setSelfUpdate (bool enable) |
enable/disable the self updating features | |
void | updateRenderWorld () |
Triggers an update of the RenderWorld object. |
Public Member Functions | |
RenderWorldWrapperWidget (QWidget *parent=NULL, Qt::WindowFlags flags=0) | |
Constructor. | |
virtual | ~RenderWorldWrapperWidget () |
Destructor. | |
virtual void | shareResourcesWith (ResourcesUser *buddy) |
Shares resources with the provided instance of ResourcesUser. | |
![]() | |
void | addUsableResource (QString resource) |
void | addUsableResources (QStringList resources) |
void | declareResource (QString name, T *resource, QString lockBuddy="") |
void | deleteResource (QString name) |
T * | getResource (QString name, bool *resourceExists=NULL) |
bool | hasResource (QString name) const |
void | removeAllUsableResources () |
void | removeUsableResource (QString resource) |
void | removeUsableResources (QStringList resources) |
void | usableResources (QStringList resources) |
bool | usedResourcesExist (QStringList *nonExistingResources=NULL) const |
Protected Member Functions | |
virtual void | customEvent (QEvent *event) |
The function that receives custom events. | |
void | lookAtRobot () |
Brings the camera in front of the robot. | |
virtual void | resourceChanged (QString resourceName, ResourceChangeType changeType) |
The function called when a resource used here is changed. | |
![]() | |
T * | getResource () |
virtual void | notifyResourceChange (ResourceHandler *resource, ResourceChangeType changeType)=0 |
ResourcesUser & | operator= (const ResourcesUser &other) |
ResourcesUser () | |
ResourcesUser (const ResourcesUser &other) |
Protected Attributes | |
QVBoxLayout *const | m_layout |
The layout for this widget. | |
RenderWorld *const | m_renderWorld |
The object actually rendering the world. | |
bool | m_renderWorldStateRestored |
True if the state of RenderWorld was restored. | |
wMatrix | m_robotTm |
The transformation matrix of the iCub. | |
QTimer * | m_selfUpdateTimer |
Timer used for self update the render world. | |
bool | m_setCameraToLookAtRobot |
True if we have to set camera to look at the robot. | |
![]() | |
ResourceCollectionHolder | m_resources |
![]() | |
ResourceCollectionHolder | m_resources |
Additional Inherited Members | |
![]() | |
typedef Resource::ResourceChangeType | ResourceChangeType |
Detailed Description
The wrapper widget for RenderWorld.
This widget contains RenderWorld. Renderworld has to be wrapped inside a QWidget, because it is not possible to set the flags as required by Total99 directly on the RenderWorld object. If you try to do this, it will crash on some windows machines :-S
Definition at line 47 of file renderworldwrapperwidget.h.
Constructor & Destructor Documentation
RenderWorldWrapperWidget | ( | QWidget * | parent = NULL , |
Qt::WindowFlags | flags = 0 |
||
) |
Constructor.
- Parameters
-
parent the parent widget flags window flags
Definition at line 57 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::m_layout, RenderWorldWrapperWidget::m_renderWorld, RenderWorldWrapperWidget::m_renderWorldStateRestored, RenderWorldWrapperWidget::m_selfUpdateTimer, RenderWorld::restoreStateFromFile(), RenderWorld::setStateFileName(), RenderWorldWrapperWidget::updateRenderWorld(), and ConcurrentResourcesUser::usableResources().
|
virtual |
Destructor.
Definition at line 88 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::m_selfUpdateTimer.
Member Function Documentation
|
protectedvirtual |
The function that receives custom events.
This function is used to receive an internal type of event that forces the update of RenderWorld. This is needed because resourceChanged is called in another thread
- Parameters
-
event the received custom event
Definition at line 146 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::updateRenderWorld().
|
slot |
return true if the self updating is active
Definition at line 123 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::m_selfUpdateTimer.
|
protected |
Brings the camera in front of the robot.
Definition at line 154 of file renderworldwrapperwidget.cpp.
References RenderWorld::camera(), RenderWorldWrapperWidget::m_renderWorld, RenderWorldWrapperWidget::m_robotTm, wMatrix::transformVector(), and wMatrix::w_pos.
Referenced by RenderWorldWrapperWidget::updateRenderWorld().
|
protectedvirtual |
The function called when a resource used here is changed.
- Parameters
-
resourceName the name of the resource that has changed. chageType the type of change the resource has gone through (whether it was created, modified or deleted)
Reimplemented from ConcurrentResourcesUser.
Definition at line 127 of file renderworldwrapperwidget.cpp.
References Logger::info(), RenderWorldWrapperWidget::m_renderWorldStateRestored, RenderWorldWrapperWidget::m_robotTm, and RenderWorldWrapperWidget::m_setCameraToLookAtRobot.
|
slot |
enable/disable the self updating features
Definition at line 115 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::m_selfUpdateTimer.
|
virtual |
Shares resources with the provided instance of ResourcesUser.
We override this function to share resources with the inner RenderWorld object
- Parameters
-
buddy the instance with which resources will be shared
- Note
- This is NOT thread safe (both this and the other instance should not be being accessed by other threads). Moreover do not call this while holding the lock to resources
Reimplemented from ConcurrentResourcesUser.
Definition at line 93 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::m_renderWorld, and ConcurrentResourcesUser::shareResourcesWith().
|
slot |
Triggers an update of the RenderWorld object.
Definition at line 102 of file renderworldwrapperwidget.cpp.
References RenderWorldWrapperWidget::lookAtRobot(), RenderWorldWrapperWidget::m_renderWorld, RenderWorldWrapperWidget::m_renderWorldStateRestored, and RenderWorldWrapperWidget::m_setCameraToLookAtRobot.
Referenced by RenderWorldWrapperWidget::customEvent(), and RenderWorldWrapperWidget::RenderWorldWrapperWidget().
Member Data Documentation
|
protected |
The layout for this widget.
Definition at line 118 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::RenderWorldWrapperWidget().
|
protected |
The object actually rendering the world.
Definition at line 113 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::lookAtRobot(), RenderWorldWrapperWidget::RenderWorldWrapperWidget(), RenderWorldWrapperWidget::shareResourcesWith(), and RenderWorldWrapperWidget::updateRenderWorld().
|
protected |
True if the state of RenderWorld was restored.
If the state of RenderWorld was not restored when we get the robot transformation matrix, we try to put the camera in front of the robot
Definition at line 126 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::RenderWorldWrapperWidget(), RenderWorldWrapperWidget::resourceChanged(), and RenderWorldWrapperWidget::updateRenderWorld().
|
protected |
The transformation matrix of the iCub.
This is used to have the camera look at the robot if needed
Definition at line 138 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::lookAtRobot(), and RenderWorldWrapperWidget::resourceChanged().
|
protected |
Timer used for self update the render world.
Definition at line 141 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::isSelfUpdate(), RenderWorldWrapperWidget::RenderWorldWrapperWidget(), RenderWorldWrapperWidget::setSelfUpdate(), and RenderWorldWrapperWidget::~RenderWorldWrapperWidget().
|
protected |
True if we have to set camera to look at the robot.
Definition at line 131 of file renderworldwrapperwidget.h.
Referenced by RenderWorldWrapperWidget::resourceChanged(), and RenderWorldWrapperWidget::updateRenderWorld().
The documentation for this class was generated from the following files:
- experiments/include/renderworldwrapperwidget.h
- experiments/src/renderworldwrapperwidget.cpp